You are working for a computer hardware store and you want to write a program that handles their price list. You are keeping your data in a dynamically allocated array of structures: struct Part { }; string partNo; string partName; double price;
You are working for a computer hardware store and you want to write a program that handles their price list. You are keeping your data in a dynamically allocated array of structures: struct Part { }; string partNo; string partName; double price;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Can you write in C++
![Question 1)
You are working for a computer hardware store and you want to write a program that handles
their price list. You are keeping your data in a dynamically allocated array of structures:
struct Part {
};
string partNo;
string partName;
double price;
Part* parts new Part [n];
Your program starts by printing a menu (a separate function) in the middle of the screen:
1. Add a new part
2. Find the price for a particular part
3. Exit
If the choice returned to main() is 1), you call another function that inputs a new part and
appends it to the array. If the choice is 2) you call another function that asks the user for a
part number, finds that part in the array, and displays the rest of the information (part name
and price). If the part number entered is not in the array, then the program displays an
appropriate message. This keeps repeating until the user select choice 3).
You will find the skeleton for the program on Moodle](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F09ff0fd5-592f-4cf4-93bb-de80ac75f898%2F6f07bfc3-95eb-4f35-9527-e4eb83b6b858%2F6adbfte_processed.png&w=3840&q=75)
Transcribed Image Text:Question 1)
You are working for a computer hardware store and you want to write a program that handles
their price list. You are keeping your data in a dynamically allocated array of structures:
struct Part {
};
string partNo;
string partName;
double price;
Part* parts new Part [n];
Your program starts by printing a menu (a separate function) in the middle of the screen:
1. Add a new part
2. Find the price for a particular part
3. Exit
If the choice returned to main() is 1), you call another function that inputs a new part and
appends it to the array. If the choice is 2) you call another function that asks the user for a
part number, finds that part in the array, and displays the rest of the information (part name
and price). If the part number entered is not in the array, then the program displays an
appropriate message. This keeps repeating until the user select choice 3).
You will find the skeleton for the program on Moodle
![#include <iostream>
#include <iomanip>
using namespace std;
struct Part {
};
/** function that displays the menu and returns the choice
* @return the choice entered by the user
*/
int menu (){
}
/** function then gets info for a new part from the user and
* appends it to the array
string partNo;
string partName;
double price;
}
// Global structure
*
*
*/
void newpart (Part* parts, int &size) {
}
/** function then asks the user for a part no. and
* finds the part in the array and returns its index
* @param parts, a pointer to the array of Parts
*
@param size, the size of the array of Parts
* @return the position of the particular part in the array,
*
returns -1 if the part is not found
*/
int find (Part* parts, int size) {
I
@param parts, a pointer to an array of Parts
@param size, the size of the array of Parts
}
/** function then prints the info relevant to a particular part
* @param parts, a pointer to the array of Parts
* @param pos, the index of the particular part
*/
void print (Part* parts, int pos) {
į
int main() {
int n = 10;
Part* parts = new Part [n]; //a dynamicaly allocated array of structures
int size = 0;
int choice;
do {
choice menu();
switch (choice) {
case 1: newpart (parts, size); break;
case 2: {int pos = find (parts, size);
if (pos
== -1) cout << "not found\n"<< endl;
else print (parts, pos); break; }
default: break;
}
} while (choice != 3);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F09ff0fd5-592f-4cf4-93bb-de80ac75f898%2F6f07bfc3-95eb-4f35-9527-e4eb83b6b858%2Fsit6x2_processed.png&w=3840&q=75)
Transcribed Image Text:#include <iostream>
#include <iomanip>
using namespace std;
struct Part {
};
/** function that displays the menu and returns the choice
* @return the choice entered by the user
*/
int menu (){
}
/** function then gets info for a new part from the user and
* appends it to the array
string partNo;
string partName;
double price;
}
// Global structure
*
*
*/
void newpart (Part* parts, int &size) {
}
/** function then asks the user for a part no. and
* finds the part in the array and returns its index
* @param parts, a pointer to the array of Parts
*
@param size, the size of the array of Parts
* @return the position of the particular part in the array,
*
returns -1 if the part is not found
*/
int find (Part* parts, int size) {
I
@param parts, a pointer to an array of Parts
@param size, the size of the array of Parts
}
/** function then prints the info relevant to a particular part
* @param parts, a pointer to the array of Parts
* @param pos, the index of the particular part
*/
void print (Part* parts, int pos) {
į
int main() {
int n = 10;
Part* parts = new Part [n]; //a dynamicaly allocated array of structures
int size = 0;
int choice;
do {
choice menu();
switch (choice) {
case 1: newpart (parts, size); break;
case 2: {int pos = find (parts, size);
if (pos
== -1) cout << "not found\n"<< endl;
else print (parts, pos); break; }
default: break;
}
} while (choice != 3);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
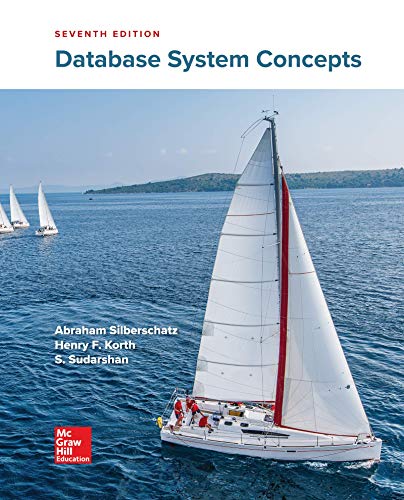
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
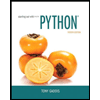
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
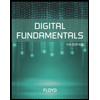
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
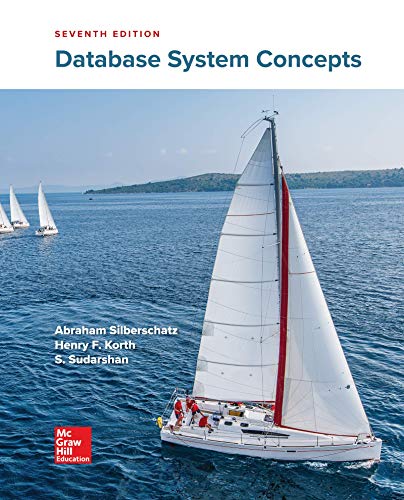
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
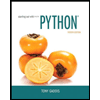
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
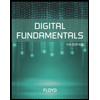
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
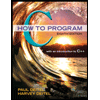
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
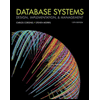
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
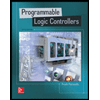
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education